Build a dApp from Scratch: Best Practices for Developers
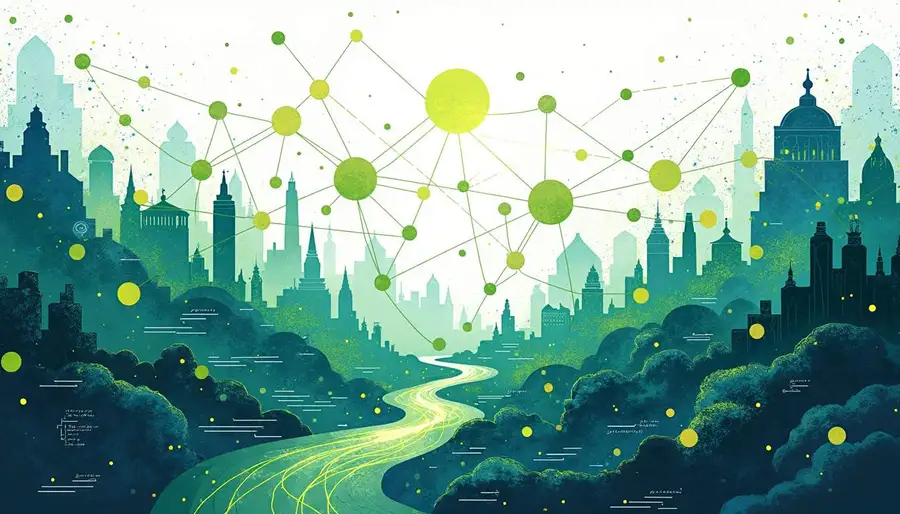
Decentralized applications, dApps, have changed the way we think about software. Unlike traditional applications, dApps run on blockchains, ensuring transparency, security, and decentralization. They power DeFi protocols for lending and trading, NFT marketplaces for digital assets, and prediction markets for real-world events, among many other Web3 innovations.
But building a functional, scalable dApp involves more than just deploying a smart contract. One of the biggest challenges? Accessing real-world data.
Blockchains are self-contained and don’t natively interact with off-chain data. This means that if your dApp relies on price feeds, real-time events, or external APIs, it needs an oracle, a system that brings real-world data onto the blockchain. If you're already feeling lost by this point, don't worry. It’s important to understand the basics before diving in. You can take a step back and start with our beginner-friendly guide on blockchain oracles to get a solid foundation before continuing.
This guide walks you through the best way to build a dApp, covering blockchain selection, smart contracts, frontends, and oracles with a special focus on Morpher Oracle, a powerful and cost-effective data solution built for EVM-compatible blockchains. By the end of this article, you will have pretty much everything you need to build your dApp from scratch.
Choosing the Right Blockchain for Your dApp
Before writing a single line of code, you need to decide which blockchain will host your dApp. Each blockchain has its own advantages and trade-offs in terms of fees, scalability, and developer tools.
Your choice of blockchain will directly impact the cost, scalability, and user experience of your dApp. Here’s a breakdown of the key factors and the best options based on your needs:
Security & Decentralization: Ethereum
If your priority is maximum security and decentralization, Ethereum is the best choice. It is the most widely used smart contract platform, with the strongest network effects, developer support, and battle-tested security.
Best for: High-value DeFi protocols, institutional applications, long-term projects that prioritize security.
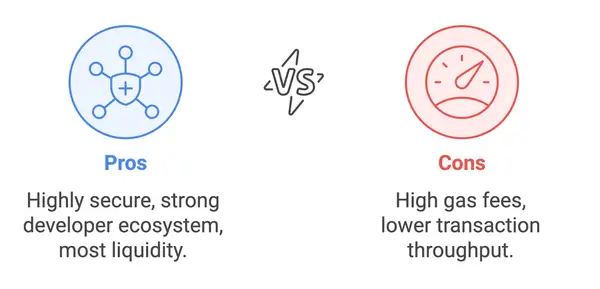
Lower Transaction Costs: Polygon & Arbitrum
If gas fees are a concern, Polygon and Arbitrum offer a more cost-effective alternative while maintaining Ethereum compatibility.
Polygon: An Ethereum Layer 2 sidechain that offers low fees and high transaction speed, making it great for NFTs, gaming, and consumer-facing dApps that require frequent interactions.
Arbitrum: A Layer 2 rollup that inherits Ethereum’s security while offering much lower gas fees. It is ideal for DeFi applications that still want to maintain the security of Ethereum.
Optimism: Another Ethereum Layer 2 rollup with a focus on cost-efficiency and developer incentives.
Best for: High-frequency trading, NFT minting, gaming, and applications needing low fees.
Binance Smart Chain: A Fast & Cheap Alternative
For projects targeting retail users with high transaction volume, Binance Smart Chain (BSC) provides fast, low-cost transactions. BSC is fully EVM-compatible, meaning it supports Solidity smart contracts, making it easy to integrate with Morpher Oracle and migrate existing Ethereum-based dApps.
Best for: Consumer dApps, gaming, retail DeFi, and applications where transaction costs must be minimal.
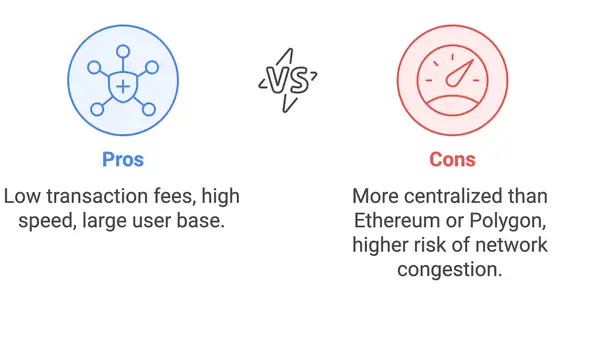
Supported EVM-Compatible Blockchains for Morpher Oracle
Morpher Oracle is designed for Ethereum and EVM-compatible blockchains, meaning it can be used seamlessly across multiple ecosystems:
Ethereum: Secure, widely used, but has high gas fees.
Polygon: Ethereum-compatible Layer 2 solution with lower fees.
Arbitrum & Optimism: Ethereum Layer 2 rollups for cost-efficient transactions.
Binance Smart Chain: Low-cost alternative with EVM compatibility.
Which One Should You Choose?
- If you are building a DeFi protocol with large TVL (Total Value Locked) and require maximum security, go with Ethereum.
- If you need scalability and lower fees while staying EVM-compatible, Polygon or Arbitrum are strong choices.
- If your dApp is targeting mass adoption, microtransactions, or consumer finance, BSC offers the lowest costs and highest speed while still being compatible with Morpher Oracle.
Regardless of your choice, Morpher Oracle is designed to work seamlessly with all EVM-compatible chains, allowing flexibility and multi-chain expansion when needed.
Core Components of a dApp
A fully functional dApp consists of three main components:
Frontend (User Interface): Built with React.js, Vue.js, or Svelte for a modern, responsive UI.
Connects to the blockchain using Ethers.js or Web3.js.
Smart Contracts (Backend on the Blockchain): Written in Solidity for Ethereum and EVM-compatible networks. Handles business logic, such as token transfers, NFT minting, or lending protocols.
Oracles (Bridging On-Chain and Off-Chain Worlds): Since smart contracts can’t directly access external data, dApps use oracles for real-world feeds.
Why Choose Morpher Oracle?
Oracles are essential for dApps that require real-time price feeds, external data verification, or off-chain information. However, not all oracles are built the same. Many traditional oracles come with high costs, latency issues, and complex integration requirements; challenges that can slow down development and increase operational expenses.
Morpher Oracle takes a smarter, more cost-efficient approach that makes life easier for developers.
- Lower Costs: Traditional oracles charge high fees for data access. Morpher Oracle is built on an ERC-4337 account abstraction model, making it cheaper and more efficient than alternatives.
- Seamless EVM Compatibility: Works natively on Ethereum, Polygon, Arbitrum, and BNB Chain, so you can deploy your dApp on multiple chains without compatibility issues.
- Data Freshness & Accuracy: Morpher Oracle uses a robust data validation system combined with an intent-based oracle model to ensure your dApp receives the most up-to-date, tamper-proof data exactly when it's needed. Instead of constantly pushing data on-chain, which can be costly and inefficient, Morpher Oracle fetches data on demand, reducing unnecessary transactions while maintaining accuracy for price feeds, market data, and other real-world inputs..
- Simplified Integration: Many oracles require complex configurations. Morpher Oracle offers plug-and-play integration with a developer-friendly SDK and clear documentation, so you can fetch real-time data with minimal setup.
For developers looking for a reliable, cost-effective, and scalable oracle solution, Morpher Oracle is the best choice, especially for dApps in DeFi, NFT pricing, prediction markets, and automated smart contract execution. If you want to discover more on how Morpher Oracle differs from traditional oracles, make sure to read our full guide by our CTO, Thomas.
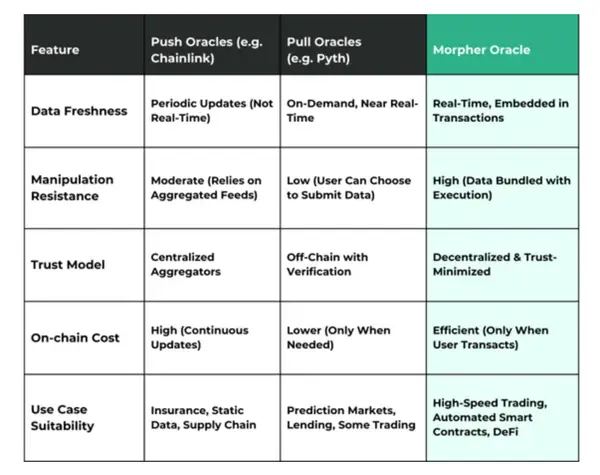
💡 Prefer to jump straight into coding? Check out the Morpher Data Feeds and start building today.
The Best Way to Build a dApp: A Step-by-Step Guide Using Morpher Oracle
Integrating Morpher Oracle into your dApp involves four key steps:
1. Implementing the DataDependent Interface
2. Calling consumeData() to fetch oracle data
3. Setting up an ERC-4337 client for transaction automation
4. Deploying and optimizing your dApp for real-time data access
Let’s go through each step.
Step 1: Implement the DataDependent Interface
To request external data, your smart contract must specify what data it needs from the oracle. This is done by implementing the DataDependent interface.
Example: Requesting BTC/USD Price Data
pragma solidity ^0.8.26;
interface DataDependent {
struct DataRequirement {
address provider;
address requester;
bytes32 dataKey;
}
function requirements(
bytes4 _selector
) external view returns (DataRequirement[] memory);
}
contract YourContract is DataDependent {
address dataProvider; //get this from the Feed page
address oracle;
bytes32 BTC_USD = keccak256("BINANCE:BTC_USDT");
// ...
function requirements(bytes4 _selector) external view override returns (DataRequirement[] memory) {
if (_selector == 0x6a627842) {
DataRequirement[] memory requirement = new DataRequirement[](1);
requirement[0] = DataRequirement(dataProvider, address(this), BTC_USD);
return requirement;
}
return new DataRequirement[](0);
}
// ...
}
What This Does:
- Defines which data provider the contract will fetch from.
- Specifies the exact data key required (BTC/USD price from Binance).
- Implements the requirements() function, so the contract knows what data to request.
Step 2: Calling consumeData() to Retrieve Oracle Data
Once your contract has defined its data requirements, it needs to fetch real-time data from the oracle when required. This is done using the consumeData() function.
Example: Fetching BTC/USD Price from the Oracle
pragma solidity ^0.8.26;
interface OracleEntrypoint {
function consumeData(
address _provider,
bytes32 _dataKey
) public payable returns (bytes32);
function prices(address _provider, bytes32 _dataKey) public view returns(uint256);
}
contract YourContract is DataDependent {
struct ResponseWithExpenses {
uint value;
uint expenses;
}
// ...
function _invokeOracle(address _provider, bytes32 _key) private returns (ResponseWithExpenses memory) {
uint expenses = OracleEntrypoint(oracle).prices(_provider, _key);
// pay the oracle now, then get the funds later from sender as you wish (eg. deduct from msg.value)
bytes32 response = OracleEntrypoint(oracle).consumeData{value: expenses}(_provider, _key);
uint256 asUint = uint256(response);
uint256 timestamp = asUint >> (26 * 8);
// in this example we want the price to be fresh
require(timestamp > 1000 * (block.timestamp - 30), "Data too old!");
uint8 decimals = uint8((asUint >> (25 * 8)) - timestamp * (2 ** 8));
// in this example we expect a response with 18 decimals
require(decimals == 18, "Oracle response with wrong decimals!");
uint256 price = uint256(
asUint - timestamp * (2 ** (26 * 8)) - decimals * (2 ** (25 * 8))
);
return ResponseWithExpenses(price, expenses);
}
// ...
}
What This Does:
- Calls consumeData() on the Morpher Oracle to fetch the latest BTC/USD price.
- Ensures the data is fresh (prevents using outdated information).
- Converts the response into a readable price value with the correct decimals.
Step 3: Setting Up an ERC-4337 Client
Now that your contract can fetch real-time oracle data, you need to ensure that users can interact with it seamlessly. ERC-4337 (Account Abstraction) helps by automating gas payments and making transactions more efficient.
Setup Instructions
- This kit will:
- Fetch your smart contract’s data requirements automatically.
- Estimate gas fees using the modified RPC endpoint.
- Submit data-dependent transactions seamlessly.
2. Use your chosen provider’s Bundler RPC to submit transactions efficiently.
Why This Matters:
- Removes the need for users to manually send transactions.
- Simplifies oracle data fetching by automating requests.
- Improves UX by making transactions gas-efficient and scalable.
Step 4: Deploying and Optimizing Your dApp
Now that everything is working, it’s time to deploy and optimize your dApp for real-world use.
Testing Before Deployment
- Deploy your smart contract to testnets before launching on mainnet:
- Ethereum Testnets: Goerli, Sepolia
- Polygon Testnet: Mumbai
- Use Hardhat or Foundry to simulate transactions before live deployment.
Optimizing for Low Gas Costs
- Use ERC-4337 account abstraction for more cost-efficient transactions.
- Optimize smart contract storage and logic to reduce gas fees.
- Batch oracle requests when possible to minimize repeated calls.
Next Steps
At this point, your dApp is ready to fetch real-time oracle data, process it within smart contracts, and execute gas-optimized transactions on Ethereum, Polygon, or BSC.
Want to see this process in action? We’ve prepared a step-by-step developer tutorial with a working NFT dApp that showcases Morpher Oracle’s full capabilities.
🎥 Watch the full tutorial here and follow along with real code examples.
What You’ll Learn in the Tutorial:
✅ Implementing the DataDependent Interface in a live smart contract.
✅ Fetching real-time price feeds using Morpher Oracle.
✅ Setting up an ERC-4337 client for automated transactions.
✅ Deploying and testing your dApp on Ethereum, Polygon, and BSC.
Ready to bring real-time data to your dApp? Let’s build something amazing!
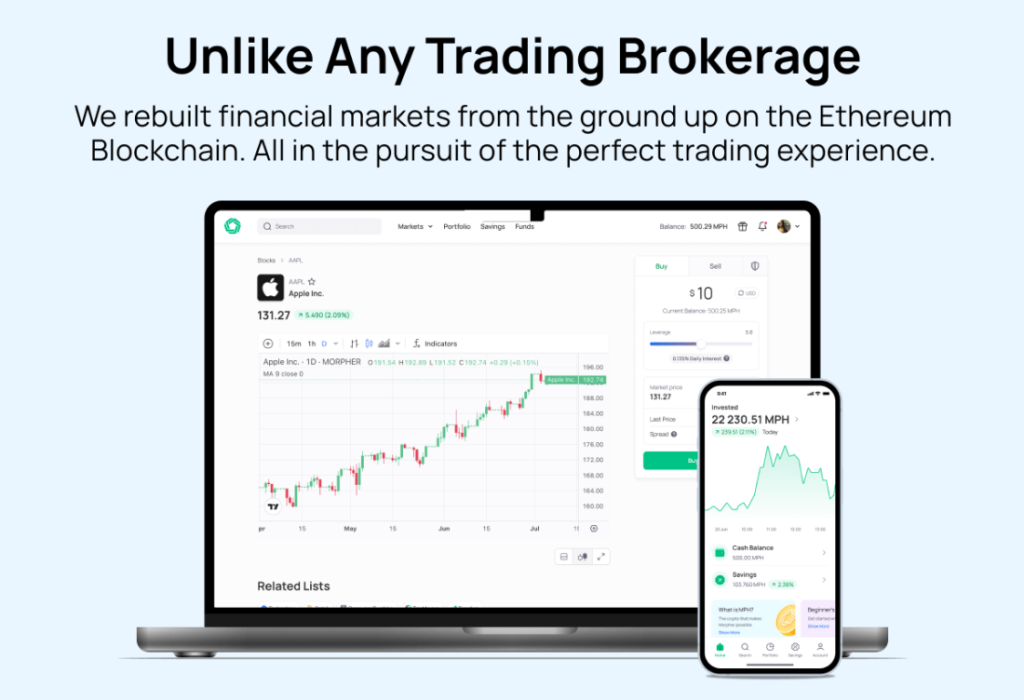
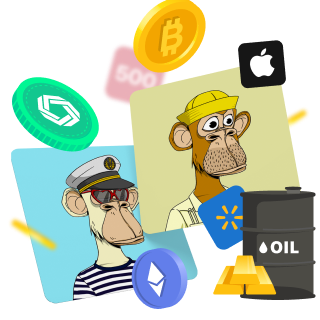
Painless trading for everyone
Hundreds of markets all in one place - Apple, Bitcoin, Gold, Watches, NFTs, Sneakers and so much more.
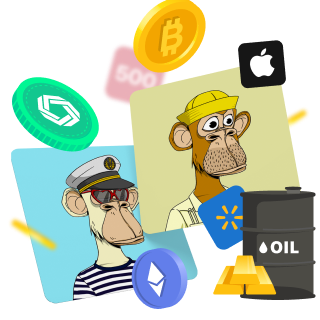
Painless trading for everyone
Hundreds of markets all in one place - Apple, Bitcoin, Gold, Watches, NFTs, Sneakers and so much more.